Effect Configuration
Utilize Effects to transform the map's visual appearance, adding a personal, artistic touch to your geospatial presentations. Find code samples for each parameters below each effect type.
Note:
This documentation is specific to managing effects from the Map SDK. To learn about how to use effects from the Studio UI, read about post-processing effects for Studio.
With a robust set of post-processing effects available, users familiar with Photoshop and other image editing programs should find a familiar set of functionality.
Usage
To add a new effect to the map, call the addEffect
(or add_effect
) function via the Map SDK.
map.addEffect({
type: "light-and-shadow",
parameters: {
shadowIntensity: 0.5,
shadowColor: [0, 0, 0],
sunLightColor: [255, 255, 255],
sunLightIntensity: 1,
ambientLightColor: [255, 255, 255],
ambientLightIntensity: 1,
timeMode: "current"
}
});
map.add_effect({
"type": "light-and-shadow",
"parameters": {
"shadowIntensity": 0.5,
"shadowColor": [0, 0, 0],
"sunLightColor": [255, 255, 255],
"sunLightIntensity": 1,
"ambientLightColor": [255, 255, 255],
"ambientLightIntensity": 1,
"timeMode": "current"
}
})
Effects can be updated in a similar fashion, but requires an ID to update. Effect IDs can be retrieved by calling map.getEffects()
/map.get_effects()
.
map.updateEffect({
id: "effect-id",
isEnabled: false,
parameters: {
shadowIntensity: 0.75,
shadowColor: [25, 50, 75]
},
});
map.update_effect({
"id": "effect-id",
"is_enabled": False,
"parameters": {
"shadowIntensity": 0.75,
"shadowColor": [25, 50, 75]
},
})
Note:
Effects are applied in top-to-bottom order. The composite image will be affected by the order in which effects are applied.
Available Effects
The following effects are currently available in Foursquare Studio. This list will grow as effects are added and updated.
Each setting below includes an example image. For reference, this is the scene used in each example without any effects.
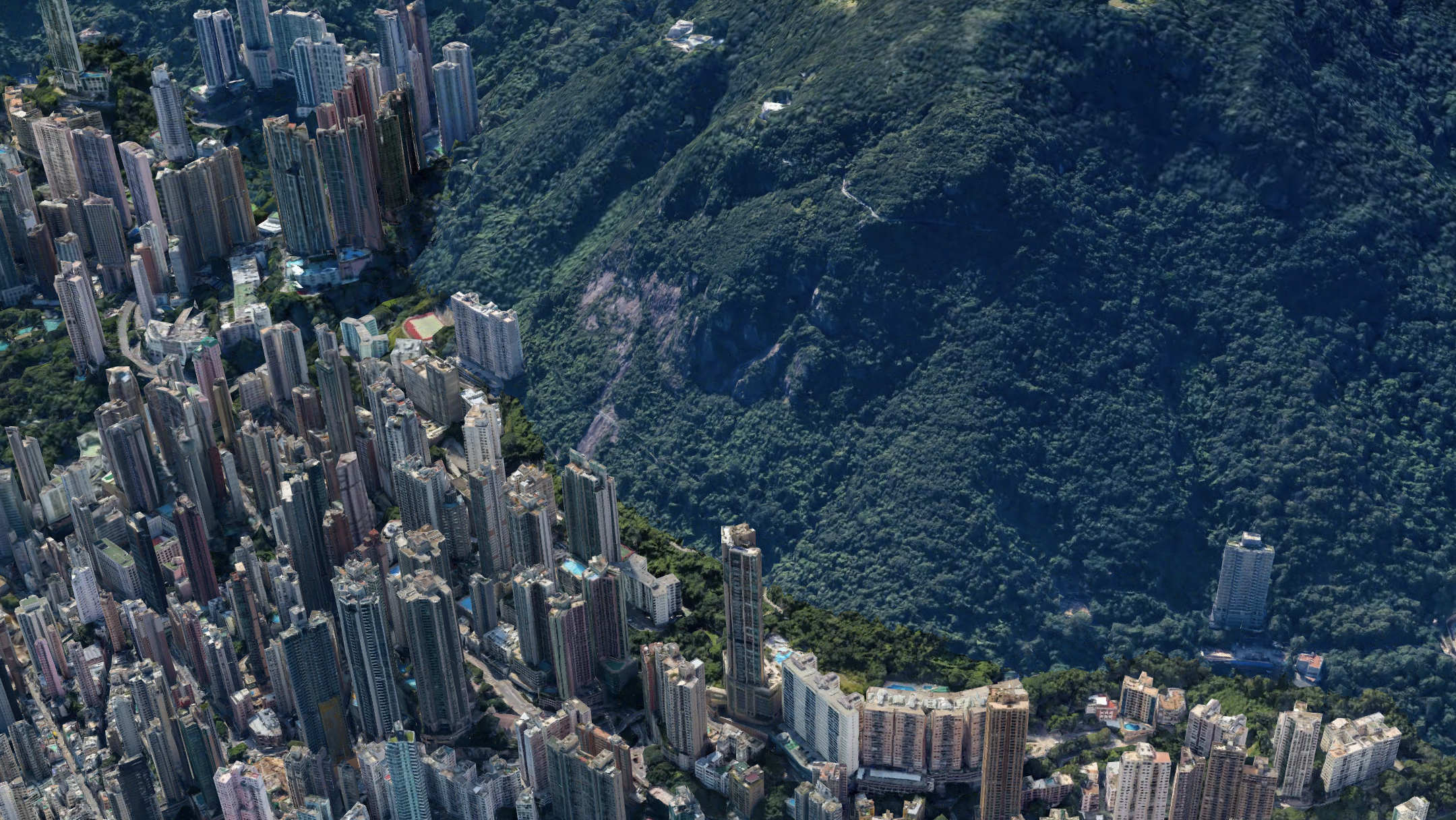
Unaltered Scene
light-shadow
Applies light and shadow effects to the map. Light, and thus shadows, are applied to 3D elements of the map, such as buildings, mountains, and any other protrusions on the earth. Light and shadows are accurately cast based on the selected time. Light and shadow intensity is fully configurable, providing for a close-to-reality map (which can be used for shadow and heat analysis), or an intense, moody composite image with unique colors and striking intensity.
Note: When the scene reaches complete ambient darkness (e.g. at nighttime) light and shadow is disabled.
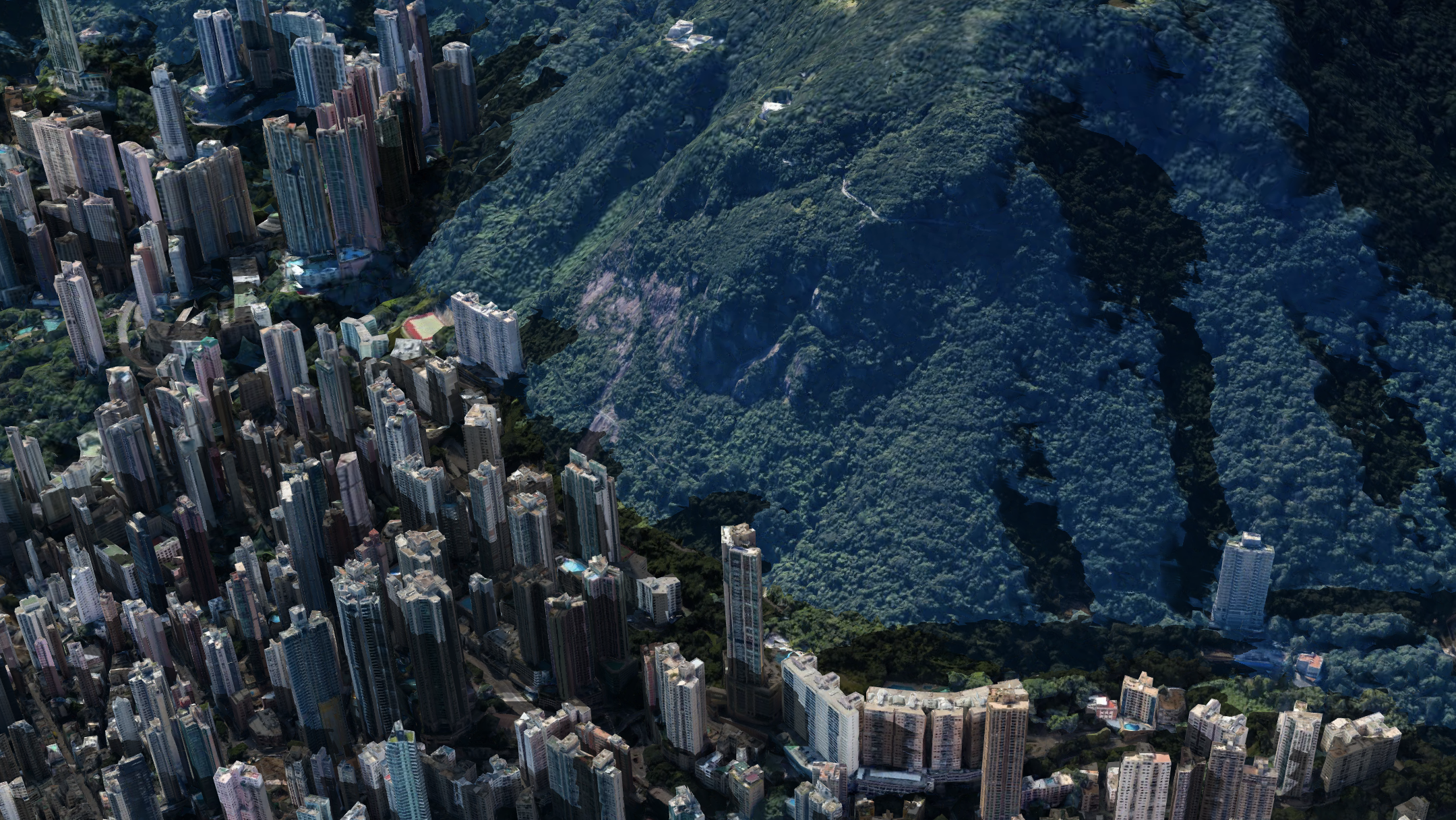
Example
map.addEffect({
type: "light-and-shadow",
parameters: {
shadowIntensity: 0.5,
shadowColor: [0, 0, 0],
sunLightColor: [255, 255, 255],
sunLightIntensity: 1,
ambientLightColor: [255, 255, 255],
ambientLightIntensity: 1,
timeMode: 'pick',
timestamp: 1695250260000
}
});
map.add_effect({
"type": "light-and-shadow",
"parameters": {
"shadowIntensity": 0.5,
"shadowColor": [0, 0, 0],
"sunLightColor": [255, 255, 255],
"sunLightIntensity": 1,
"ambientLightColor": [255, 255, 255],
"ambientLightIntensity": 1,
"timeMode": 'pick',
"timestamp": 1695250260000
}
})
light-shadow
effect parameters:
Parameter | Type | Description |
---|---|---|
shadowIntensity | number | The strength of shadows cast by 3D objects. 0 disables shadows entirely while 1 draws a completely opaque shadow. Default: 0.5 |
shadowColor | int array | The color of the shadows represented by a 3-digit hex code. Default: [0, 0, 0] |
sunLightColor | int array | The color of the sun light represented by a 3-digit hex code. Default: [255, 255, 255] |
sunLightIntensity | number | The strength of the sunlight from 0 to 1 . Default: 1 |
ambientLightColor | int array | The color of the ambient light (light artificially added to the map). Default: [255, 255, 255] |
timemode | string | The method by which to select solar time. One of pick (allowing user to select time), current (selecting the current time on Earth at the time the map is rendered), or |
timestamp | number /timestamp | Available when timemode = pick . A unix time timestamp to use for the solar time. |
timezone | string | Available when timemode = pick . Since unix time is required, selecting a timezone only updates the local time in Studio's UI, and does not effect the map. |
tilt-shift
Simulates the shallow depth of field normally encountered in close-up photography, which makes the scene seem much smaller than it actually is. This filter assumes the scene is relatively planar, in which case the part of the scene that is completely in focus can be described by a line (the intersection of the focal plane and the scene).
Example
map.addEffect({
type: "tilt-shift",
parameters: {
blurRadius: 20
}
});
map.add_effect({
"type": "tilt-shift",
"parameters": {
"blurRadius": 20
}
})
tilt-shift
effect parameters:
Setting | Type | Description |
---|---|---|
blurRadius | number | The maximum radius of the blur in pixels. Default: 15 . |
gradientRadius | number | The distance in pixels from the line at which the maximum blur radius is reached. Default: 200 . |
start | number array | [x, y] coordinate of the start of the line segment. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default: [0, 0] . |
end | number array | [x, y] coordinate of the end of the line segment. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default value is [1, 1] . |
triangle-blur
The most basic blur filter, which convolves the image with a pyramid filter. The pyramid filter is separable and is applied as two perpendicular triangle filters.
map.addEffect({
type: "triangle-blur",
parameters: {
radius: 30
}
});
map.add_effect({
"type": "triangle-blur",
"parameters": {
"radius": 30
}
})
triangle-blur
effect parameters:
Setting | Type | Description |
---|---|---|
radius | number | The maximum radius of the blur in pixels. Default: 20 . |
zoom-blur
Blurs the image away from a certain point, effectively creating a radial motion blur.
map.addEffect({
type: "zoom-blur",
parameters: {
strength: 0.5
}
});
map.add_effect({
"type": "zoom-blur",
"parameters": {
"strength": 0.5
}
})
zoom-blur
effect paramteres:
Setting | Type | Description |
---|---|---|
strength | number | The strength of the blur. Values in the range 0 to 1 are usually sufficient, where 0 doesn't change the image and 1 creates a highly blurred image. Default: 0.3 . |
center | number array | [x, y] coordinate of the blur origin. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default: [0.5, 0.5] . |
brightness-contrast
Provides additive brightness and multiplicative contrast control.
map.addEffect({
type: "brightness-contrast",
parameters: {
brightness: 0.5
}
});
map.add_effect({
"type": "brightness-contrast",
"parameters": {
"brightness": 0.5
}
})
brightness-contrast
effect parameters:
Setting | Type | Description |
---|---|---|
brightness | number | The brightness of the image from -1 to 1 , where -1 is solid black, 0 is no change, and 1 is solid white. Default: 0 . |
contrast | number | The contrast of the image from -1 to 1 , where -1 is solid gray, 0 is no change, and 1 is maximum contrast. Default: 0 . |
color-halftone
Simulates a CMYK halftone rendering of the image by multiplying pixel values with four rotated 2D sine wave patterns, one each for cyan, magenta, yellow, and black.
map.addEffect({
type: "color-halftone",
parameters: {
size: 2
}
});
map.add_effect({
"type": "color-halftone",
"parameters": {
"size": 2
}
})
color-halftone
effect parameters:
Setting | Type | Description |
---|---|---|
angle | number | The rotation of the pattern in radians. Default: 1.1 . |
size | number | The diameter of a dot in pixels. Default value is 4 . |
center | number array | [x, y] coordinate of the pattern origin. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default: [0.5, 0.5] . |
dot-screen
Simulates a black and white halftone rendering of the image by multiplying pixel values with a rotated 2D sine wave pattern.
map.addEffect({
type: "dot-screen",
parameters: {
size: 2
}
});
map.add_effect({
"type": "dot-screen",
"parameters": {
"size": 2
}
})
dot-screen
effect parameters:
Setting | Type | Description |
---|---|---|
angle | number | The rotation of the pattern in radians. Default: 1.1 . |
size | number | The diameter of a dot in pixels. Default value is 4 . |
center | number array | [x, y] coordinate of the pattern origin. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default: [0.5, 0.5] . |
edge-work
Picks out different frequencies in the image by subtracting two copies of the image blurred with different radii.
map.addEffect({
type: "edge-work",
parameters: {
radius: 0.5
}
});
map.add_effect({
"type": "edge-work",
"parameters": {
"radius": 0.5
}
})
edge-work
effect parameters:
Setting | Type | Description |
---|---|---|
radius | number | The radius of the effect in pixels. Default: 2 . |
hexagonal-pixelate
Renders the image using a pattern of hexagonal tiles. Tile colors are nearest-neighbor sampled from the centers of the tiles.
map.addEffect({
type: "hexagonal-pixelate",
parameters: {
scale: 5
}
});
map.add_effect({
"type": "hexagonal-pixelate",
"parameters": {
"scale": 5
}
})
hexagonal-pixelate
effect parameters:
Setting | Type | Description |
---|---|---|
center | number array | [x, y] coordinate of the pattern origin. [0, 0] is the bottom left corner, [1, 1] is the up right corner. Default: [0.5, 0.5] . |
scale | number | The width of an individual tile in pixels. Default: 10 . |
hue-saturation
Provides rotational hue and multiplicative saturation control. RGB color space can be imagined as a cube where the axes are the red, green, and blue color values.
Hue changing works by rotating the color vector around the grayscale line, which is the straight line from black (0, 0, 0) to white (1, 1, 1).
Saturation is implemented by scaling all color channel values either toward or away from the average color channel value.
map.addEffect({
type: "hue-saturation",
parameters: {
hue: 0.5
}
});
map.add_effect({
"type": "hue-saturation",
"parameters": {
"hue": 0.5
}
})
hue-saturation
effect parameters:
Setting | Type | Description |
---|---|---|
hue | number | Hue of the image, from -1 to 1 . -1 is 180 degree rotation in the negative direction, 0 is no change, and 1 is 180 degree rotation in the positive direction. Default: 0 . |
saturation | number | Saturation of the image from -1 to 1 . -1 is solid gray, 0 is no change, and 1 is maximum contrast. Default: 0 . |
ink
Simulates outlining the image in ink by darkening edges stronger than a certain threshold. The edge detection value is the difference of two copies of the image, each blurred using a blur of a different radius.
map.addEffect({
type: "ink",
parameters: {
strength: 0.5
}
});
map.add_effect({
"type": "ink",
"parameters": {
"strength": 0.5
}
})
ink
effect parameters:
Setting | Type | Description |
---|---|---|
strength | number | The multiplicative scale of the ink edges. Values in the range 0 to 1 are usually sufficient, where 0 doesn't change the image and 1 adds many black edges. Negative strength values will create white ink edges instead of black ones. Default: 0.25 . |
magnify
Apply magnify effect to the surrounding area of a given position. This can be used to draw attention to a specific location or element of the map.
map.addEffect({
type: "magnify",
parameters: {
screenXY: [0.5, 0.5]
}
});
map.add_effect({
"type": "magnify",
"parameters": {
"screenXY": [0.5, 0.5]
}
})
magnify
effect parameters:
Setting | Type | Description |
---|---|---|
screenXY | number array | [x, y] position in screen coords, both x and y is normalized and in range [0, 1] . [0, 0] is the up left corner, [1, 1] is the bottom right corner. Default: [0, 0] . |
radiusPixels | number | Effect radius in pixels. Default: 200 . |
zoom | number | Magnify level. Default: 2 . |
borderWidthPixels | number | The width of the border in pixels. |
noise
Adds black and white noise to the image.
map.addEffect({
type: "noise",
parameters: {
amount: 0.5
}
});
map.add_effect({
"type": "noise",
"parameters": {
"amount": 0.5
}
})
noise
effect parameters:
Setting | Type | Description |
---|---|---|
amount | number | The amount of noise on the image, from 0 to 1 (0 for no effect, 1 for maximum noise). Default: 0.5 . |
sepia
Gives the image a reddish-brown monochrome tint that imitates an old photograph.
map.addEffect({
type: "sepia",
parameters: {
amount: 0.5
}
});
map.add_effect({
"type": "sepia",
"parameters": {
"amount": 0.5
}
})
sepia
effect parameters:
Setting | Type | Description |
---|---|---|
amount | number | Amount of sepia coloring applied to the image, from 0 to 1 (0 for no effect, 1 for full sepia coloring). Default: 0.5 . |
vibrance
Modifies the saturation of desaturated colors, leaving saturated colors unmodified.
map.addEffect({
type: "vibrance",
parameters: {
amount: 0.5
}
});
map.add_effect({
"type": "vibrance",
"parameters": {
"amount": 0.5
}
})
vibrance
effect parameters:
Setting | Type | Description |
---|---|---|
amount | number | Amount of vibrance added to the image, from -1 to 1 (-1 is minimum vibrance, 0 is no change, and 1 is maximum vibrance). Default: 0 . |
vignette
Adds a simulated lens edge darkening effect.
map.addEffect({
type: "vignette",
parameters: {
amount: 0.5
}
});
map.add_effect({
"type": "vignette",
"parameters": {
"amount": 0.5
}
})
vignette
effect parameters:
Setting | Type | Description |
---|---|---|
radius | number | The radius of the vignette effect, from 0 to 1 . 0 applies no vignetting, 0.5 creates a blended vignette, and 1 creates a solid vignette. Default: 0.5 . |
amount | number | The amount of the frame to apply vignette to, from 0 to 1 . 0 applies no vignette effect, 0.5 applies a standard vignette effect, and 1 applies a maximum vignette size to the image. Default: 0.5 . |
Gallery
Below are a selection of maps utilizing one or more effects. All of these examples were made by members the Studio team. These images were completely made in Studio and were not touched by any image processing tools.
If you made a map with effects that you feel proud of, feel free to share them to our Community Slack.
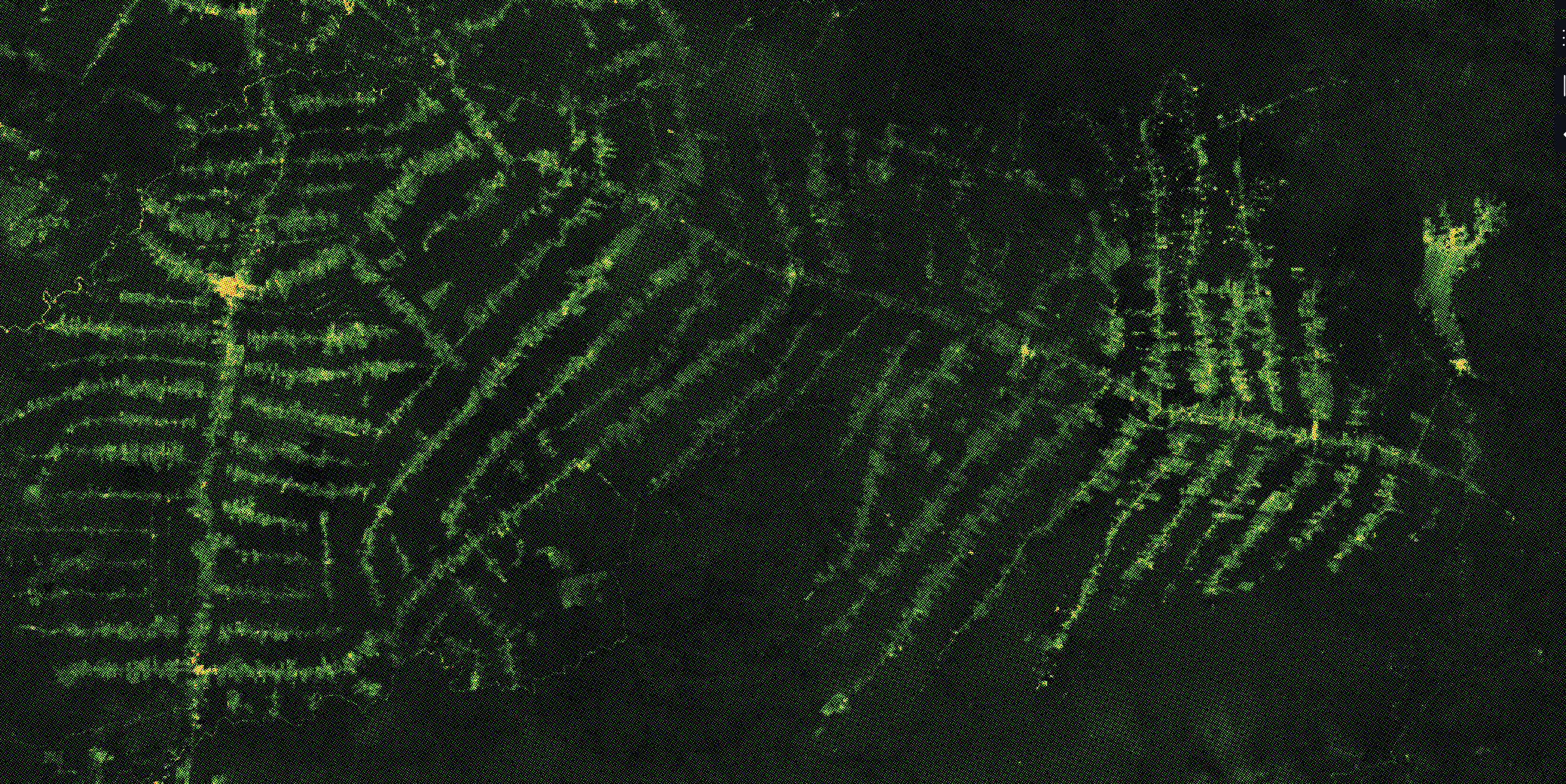
Amazon deforestation raster imagery with a color halftone.
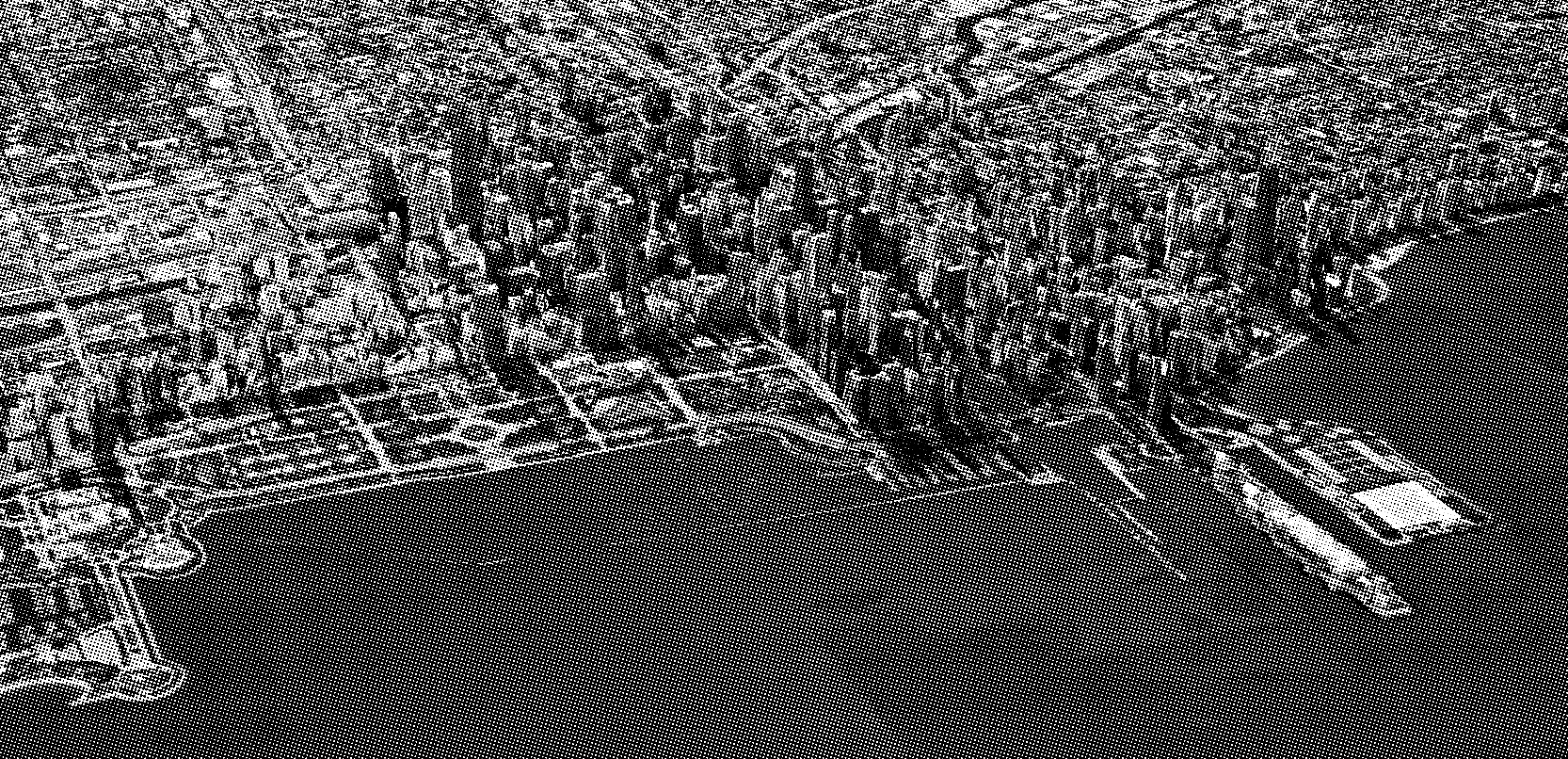
Chicago from far, represented by Google 3D tiles, light and show, and a dot screen effect.
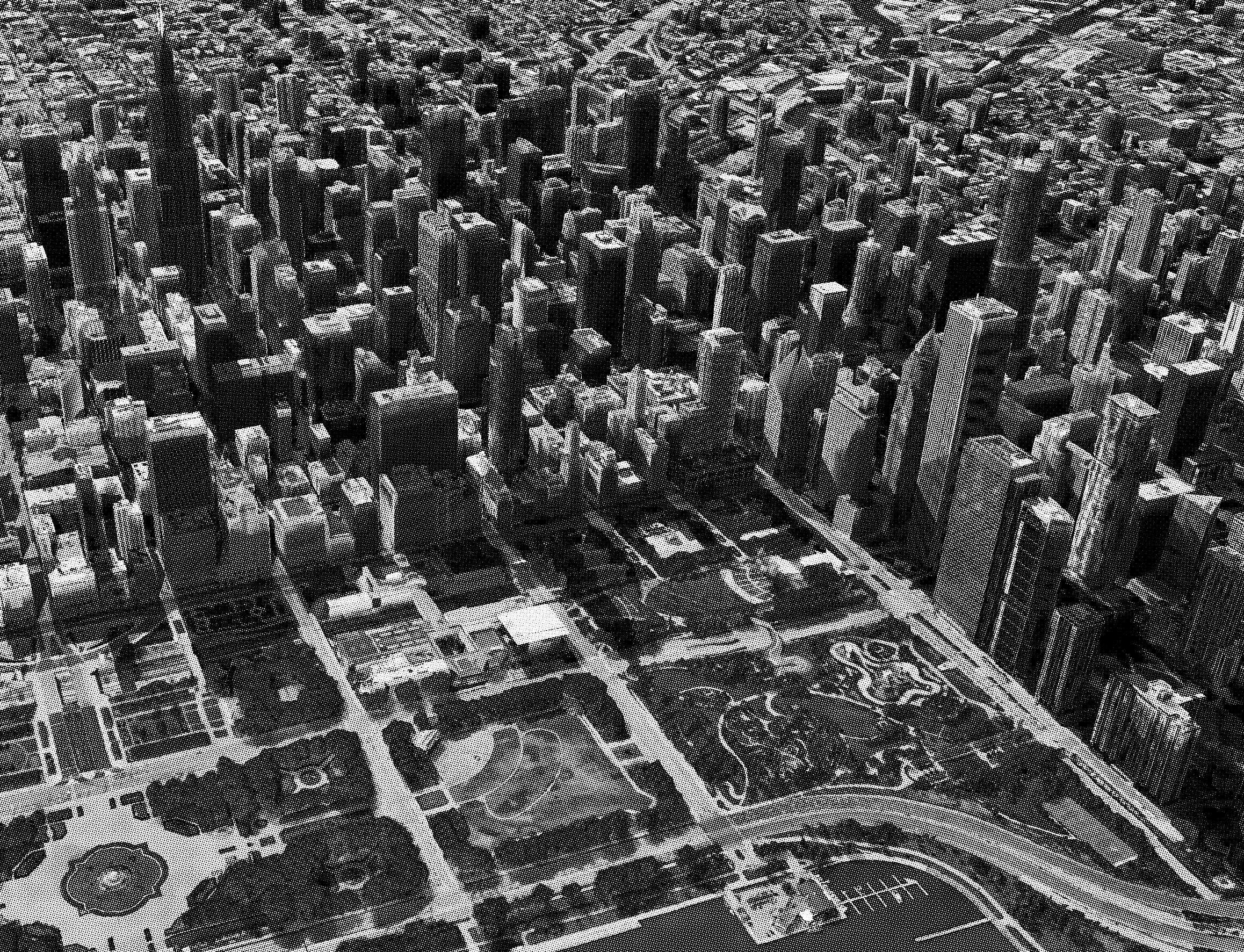
Chicago represented by Google 3D tiles, light and show, and a dot screen effect.
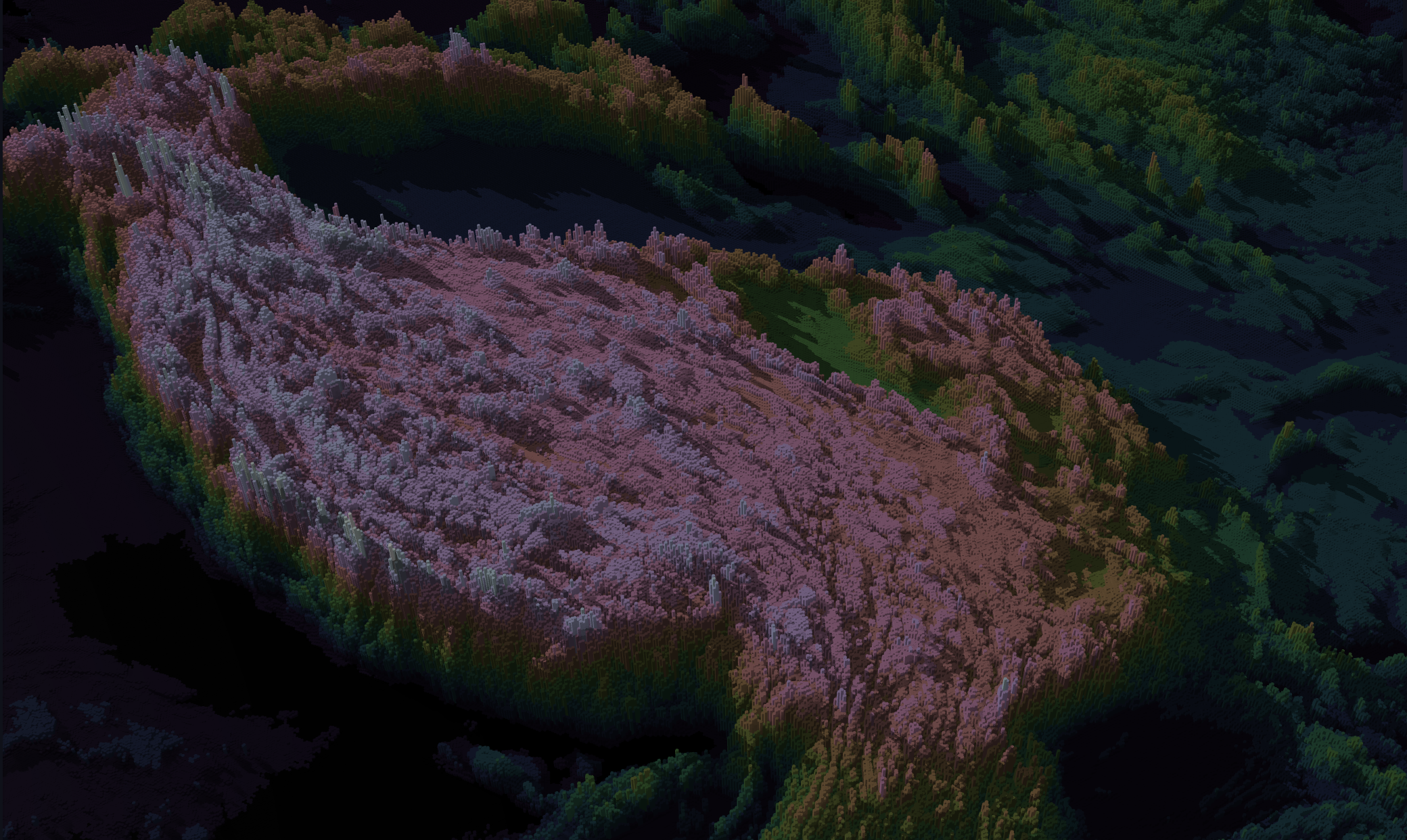
China elevation represented by Hex Tiles with a light and shadow effect.
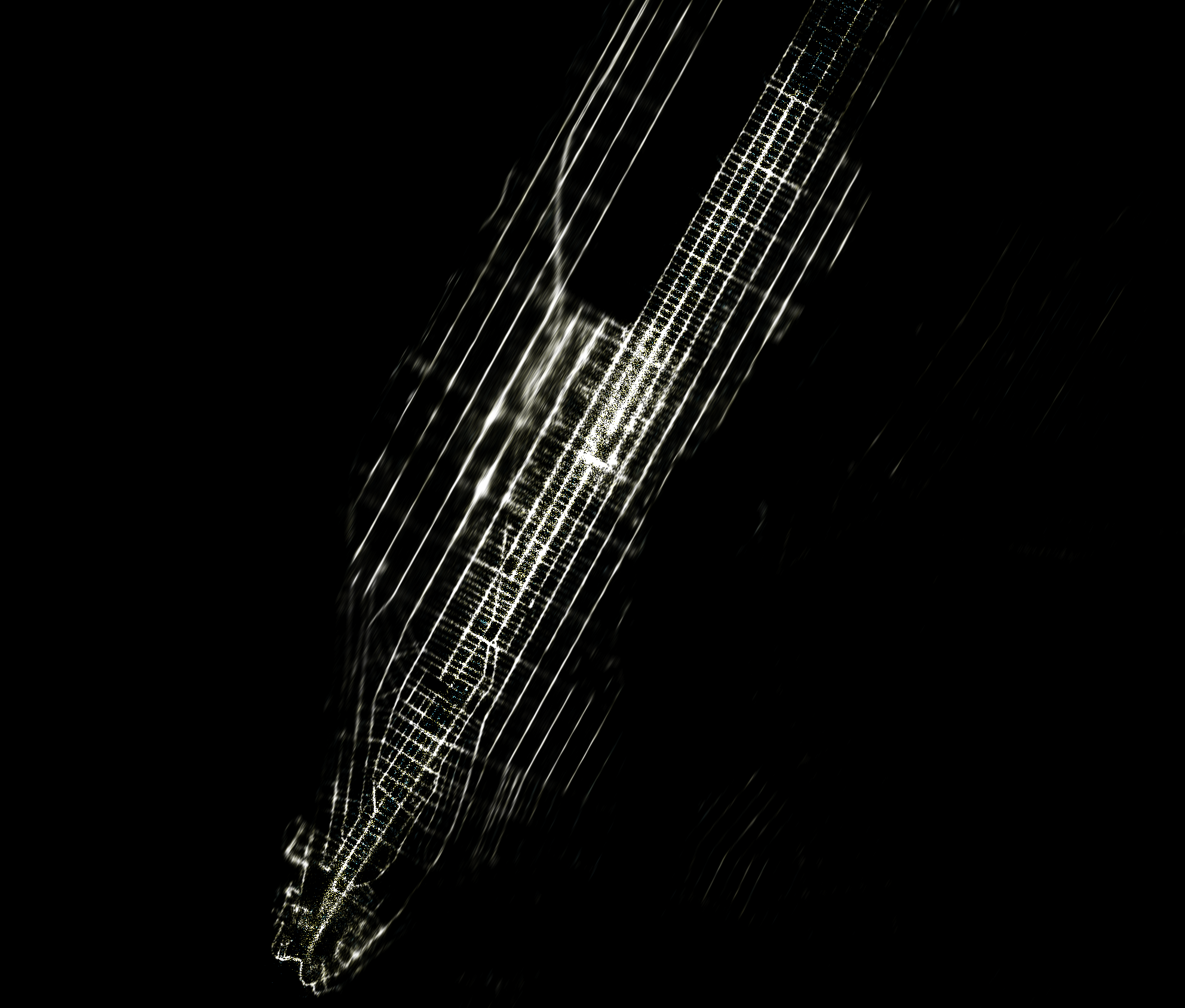
FSQ Manhattan visits with a tilt blur highlighting Fifth Avenue.
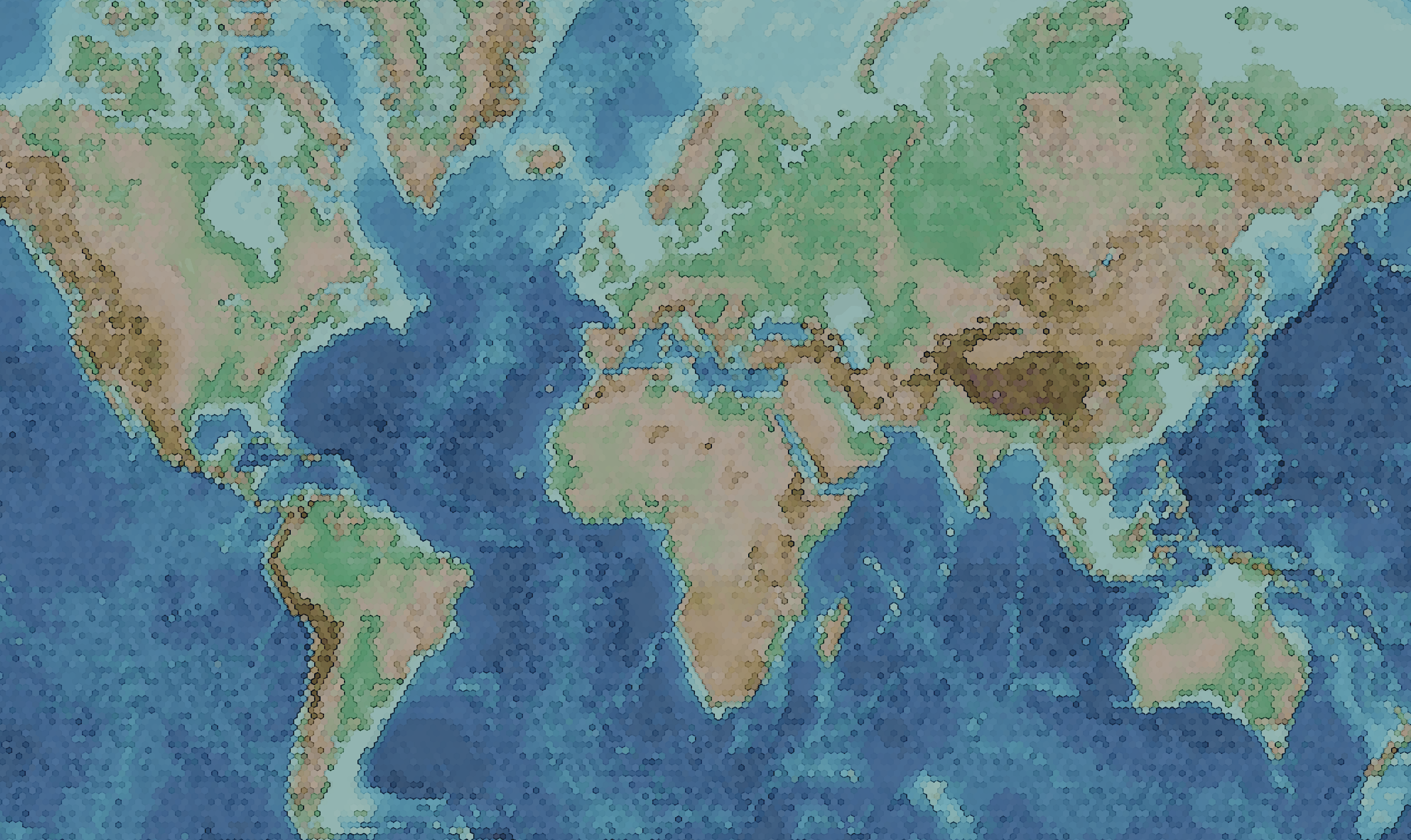
Earth with a hexagonal pixelate effect applied.
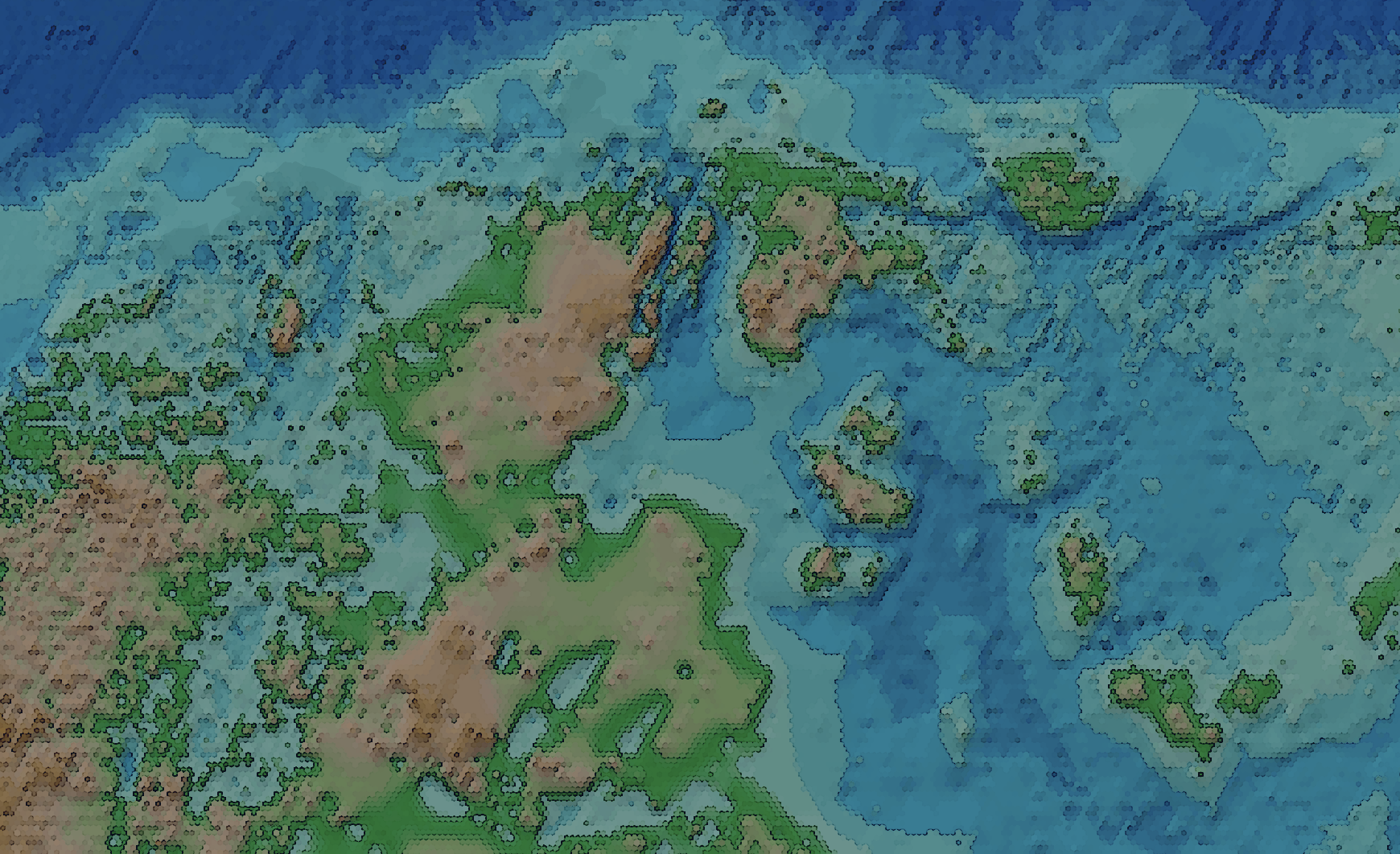
Landmass below Antartica with a hexagonal pixelate effect applied.
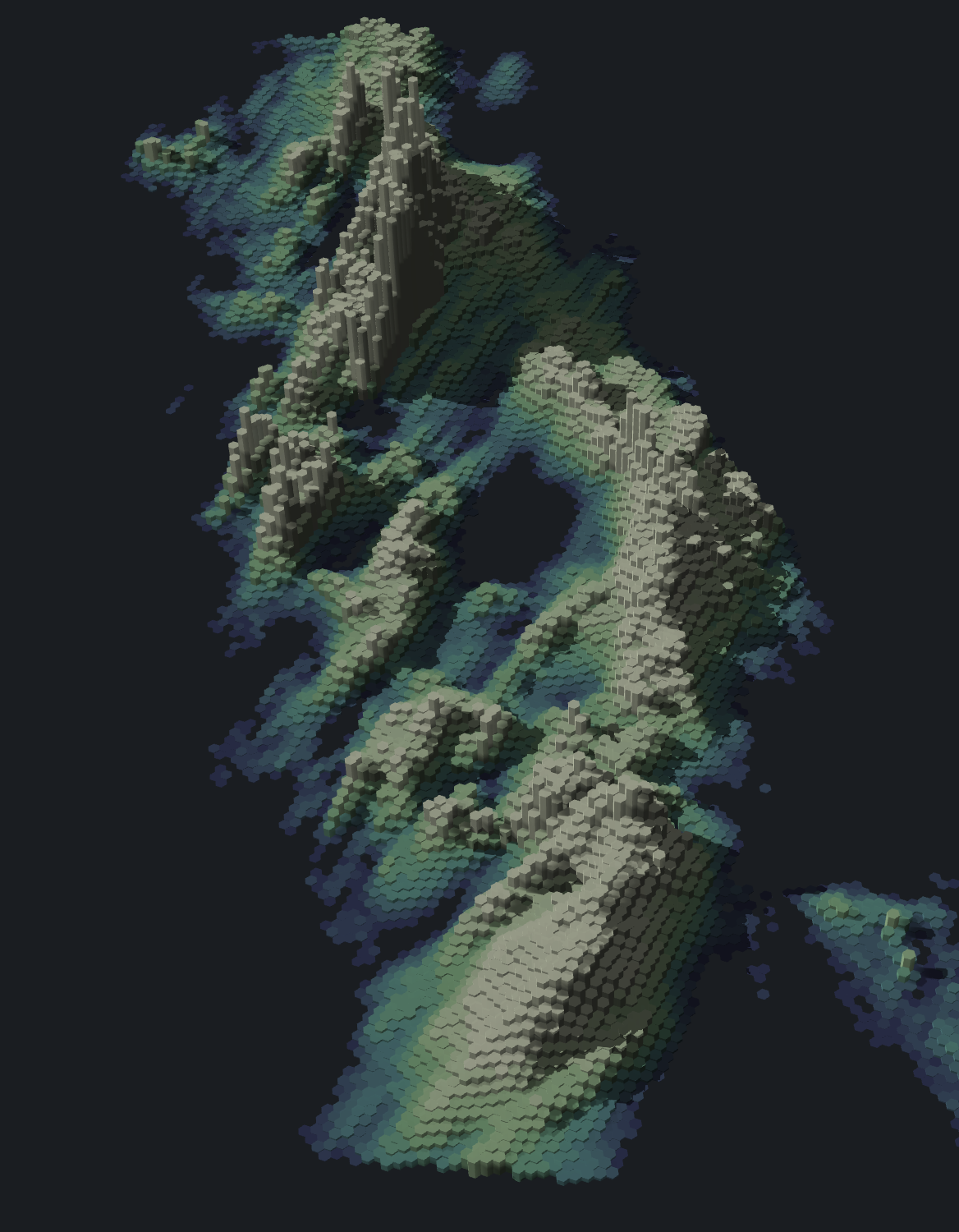
California rainstorm Hex Tiles with light and shadow applied.
Updated 3 months ago