Introduction
This document details generating access tokens that can be used by your web clients to make secure, direct calls to Foursquare Targeting’s APIs. Using tokens enables you to avoid proxying API calls through your own servers and is a required piece of implementing an embeddable UI workflow.
Requirements
The basic requirements to implement tokens to access Foursquare Targeting are:
- A server in your control that can authenticate your clients against your own user DB
- A Foursquare Targeting API key
- The ability for your server to make an outbound API call to Foursquare Targeting
- The ability for your server to forward the results of an API call from Foursquare Targeting to your clients
The diagram below details the basic authentication flow:
As shown in the diagram above, the basic steps are:
- Your user attempts to login from one of your clients by sending login credentials to your server.
- Assuming the user has acceptable credentials, your server will either:
- Return the most recent Foursquare Targeting authentication token it has for the user (assuming that it hasn’t passed its expiration date).1
- Request a new token from Foursquare Targeting on behalf of that user.2
- In the event a token was requested, Foursquare Targeting will return the token to your server.
- Your server in turn will return the access token to the client.
- Your client will use the token to make a request to Foursquare Targeting.
- Foursquare Targeting will determine if the token is valid, and what permissions it should have.
- As appropriate, Foursquare Targeting will return data to the client.
Note:
Until your client’s Foursquare Targeting token has expired, your client does not need to re-request tokens to make calls to Foursquare Targeting.
There is no harm in asking for a token redundantly. Foursquare Targeting will consistently return the same token is for that user.
If this is the first time the user has requested access to Foursquare Targeting, all necessary configuration on Foursquare Targeting’s side will be performed immediately, in order to respond to your server’s request in a timely manner. I.e., each user’s Foursquare Targeting credentials are generated quickly but lazily.
Getting an API Key
If you haven't already, contact Foursquare Targeting to register an account. Once you have an account, ask Foursquare Targeting to create a new Server App key for you.
- Go to the Foursquare Targeting API key manager at https://accounts.targeting.foursquare.com/keys.
- View your Server App key and click the Modify link.
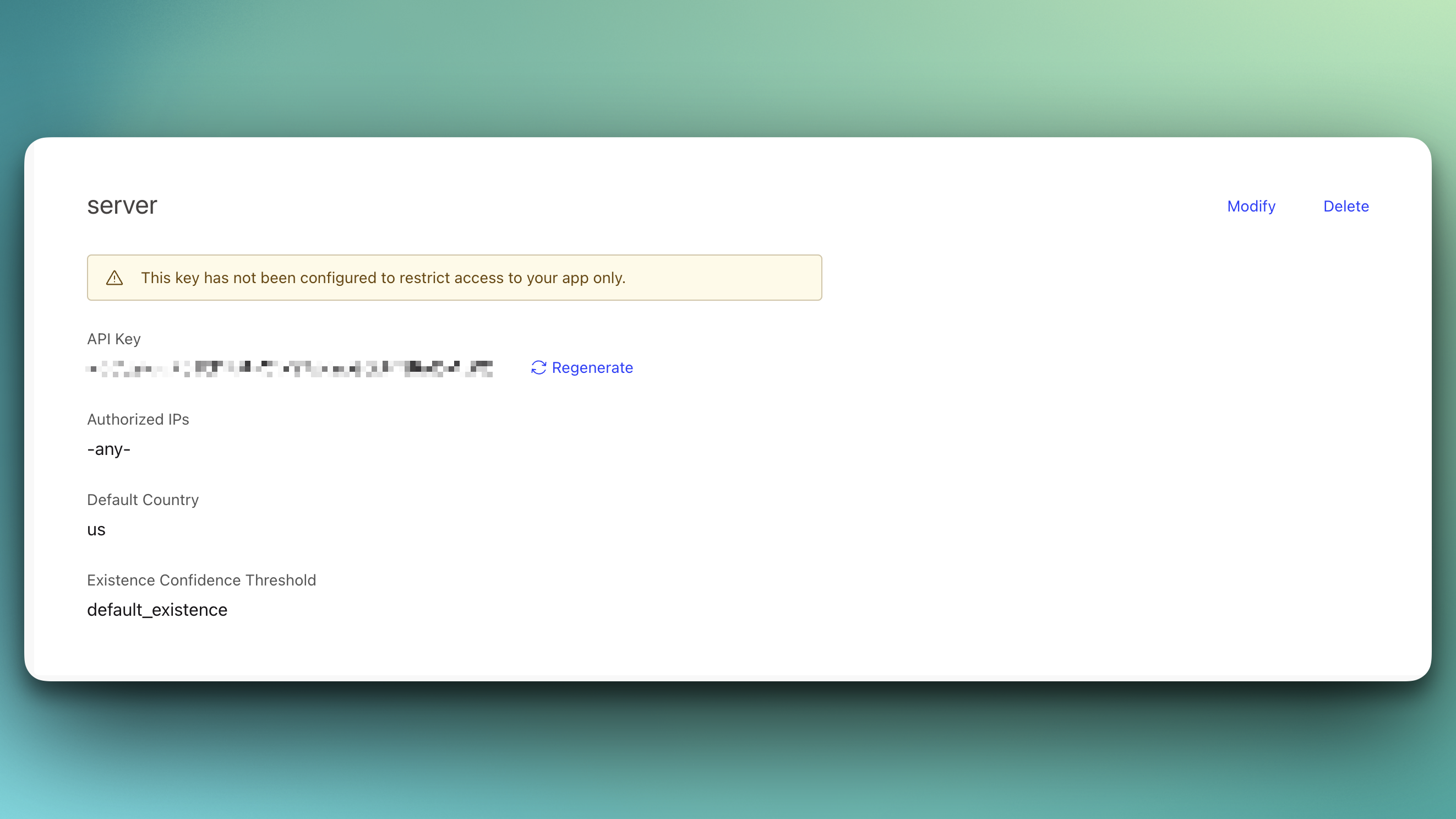
Accessing existing API keys from the key manager page.
- In the Security and Permissions section, enter the IP address of your server. This helps prevent use of your API key to generate tokens from unauthorized domains, if it were to be compromised.
- Hit Save.
Getting Tokens
Tokens are generated using an https request to Foursquare Targeting’s V4 API. Authentication of your token API call is performed simply by supplying your API key to the KEY parameter (all CAPS) to the /token/user endpoint. Along with your API key, the following parameters are required (unless you provide a user Id, as detailed later):
- email (User email address.)
- first_name (first name of user. must not be blank or all white space.)
- last_name (last name of user. must not be blank or all white space.)
Token API Request
The basic syntax for getting a token is to make a GET request as follows:
https://api.targeting.foursquare.com/token/user?email=email_address&first_name=first_name&last_name=last_name&KEY=api_key
- As per any http GET request parameter, the email address and first/last names must be URL-encoded.
- The request must be transmitted securely through https.
Refresh Token API Request
Once you have received a successful token response containing a user id, you should request future tokens for that user simply using the user id:
https://api.targeting.foursquare.com/token/user/user_id?KEY=api_key
Token API Response
Responses to the Foursquare Targeting API contain a JSON object in the response body, in addition to metadata in the http headers and the http status code. The standard http response body will contain data conforming to the JSend convention, augmented with a few other attributes:
- status (success, fail, error)
- data (for success_/_fail)
- message (for error)
- version (API version)
Standard Response
An http 200 response will be accompanied with JSON, similar to the following, containing the user’s userId, token and the expiration date of the token:
{
"version": 4,
"status": "success",
"data": {
"uid": "fbd96e3a-1d7d-4a93-b201-020e53e65417",
"token": "XTH4sYzIjP5cG6RzqSoi",
"expiration": 1443650290
}
}
You should forward both the token and expiration date to your clients. The expiration date will be in epoch time, in seconds.
Exceptional Paths
HTTP Response Code | Message | Explanation |
---|---|---|
400 | unknown parameter ‘parameter_name’ | You have entered a parameter that the API does not recognize. |
400 | parameter ‘parameter_name’ should not be used alongside a user id. | You have provided both a user id (in the URL) as well as user identifying information such as email, first_name, or last_name. You should only be providing the user Id if you have it. |
400 | missing required parameter ‘parameter_name’ | You have not provided a user id and any of the email, first_name or last_name parameters is either missing or blank. |
400 | invalid email address | The email address you provided is invalid. |
401 | invalid API key | Your API key is not valid. This is most likely because you’ve either regenerated your API key (invalidating the existing one), or because you’ve made a copy/paste mistake. |
403 | throttled | You’ve made too many API calls over the allotted period of time. |
404 | resource not found | There is a typo somewhere in the request URL. |
404 | user not found | The user id provided in the URL is invalid. |
Using Tokens
Once your client has a token and expiration date, it can make API requests directly to Foursquare Targeting. In order to make requests to Foursquare Targeting:
- Ensure that the expiration date for the token has not passed. If it has, your client-side code should be prepared to ask your server for a new token, which it will have to fetch from Foursquare Targeting.
- Insert the valid token into the Authorization header as an OAuth 2.0 Bearer token.
For example, if the token is XTH4sYzIjP5cG6RzqSoi, the header would look like:
GET /geopulse/designs/ HTTP/1.1
Host: api.targeting.foursquare.com
Authorization: Bearer XTH4sYzIjP5cG6RzqSoi
Refreshing Tokens
If the token has expired, your server simply needs to make a new request to the token API with the appropriate user id. Foursquare Targeting will return a new token.