Building a local search map can be easier than you think. We’ll be walking through how to do so by using simple place search autocomplete inputs and Vanilla Javascript. In this example, we’ll be using and referencing the Foursquare Places API and Mapbox, which is available to try for free by creating a Foursquare account.
Getting started
Here’s what we’ll need:
- JavaScript
- Foursquare API token
- Mapbox API token
- HTML for the search map
- CSS for styling
- Note that we have not included the CSS styling here, but our full Local Search Map demo includes CSS styling for reference.
1 . Create a simple HTML template and import Mapbox gl for rendering the map.
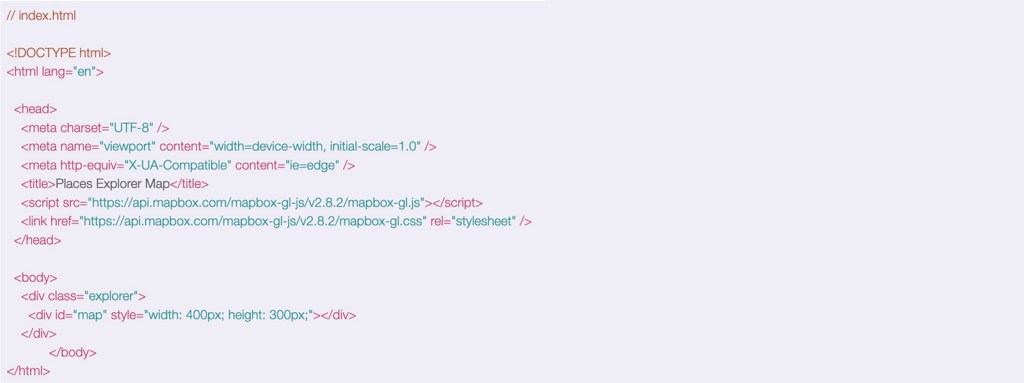
2 . On the JavaScript file or the < script> tag in the HTML file, initialize the Mapbox map. (For more advance usage, check out Mapbox’s official documentation).
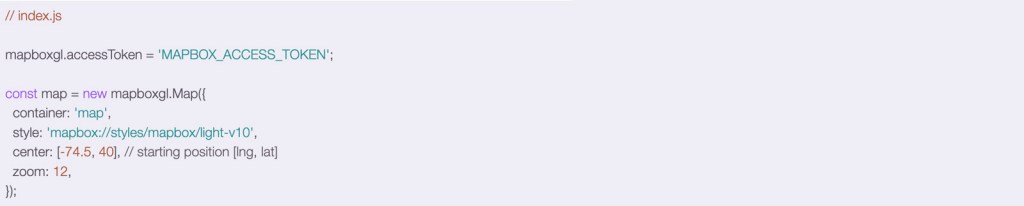
3 . Next, add our search box to the HTML.

The explorer-suggestions will be used for the list of places results.
4 . Let’s add a method that triggers a call to the Foursquare Autocomplete API.
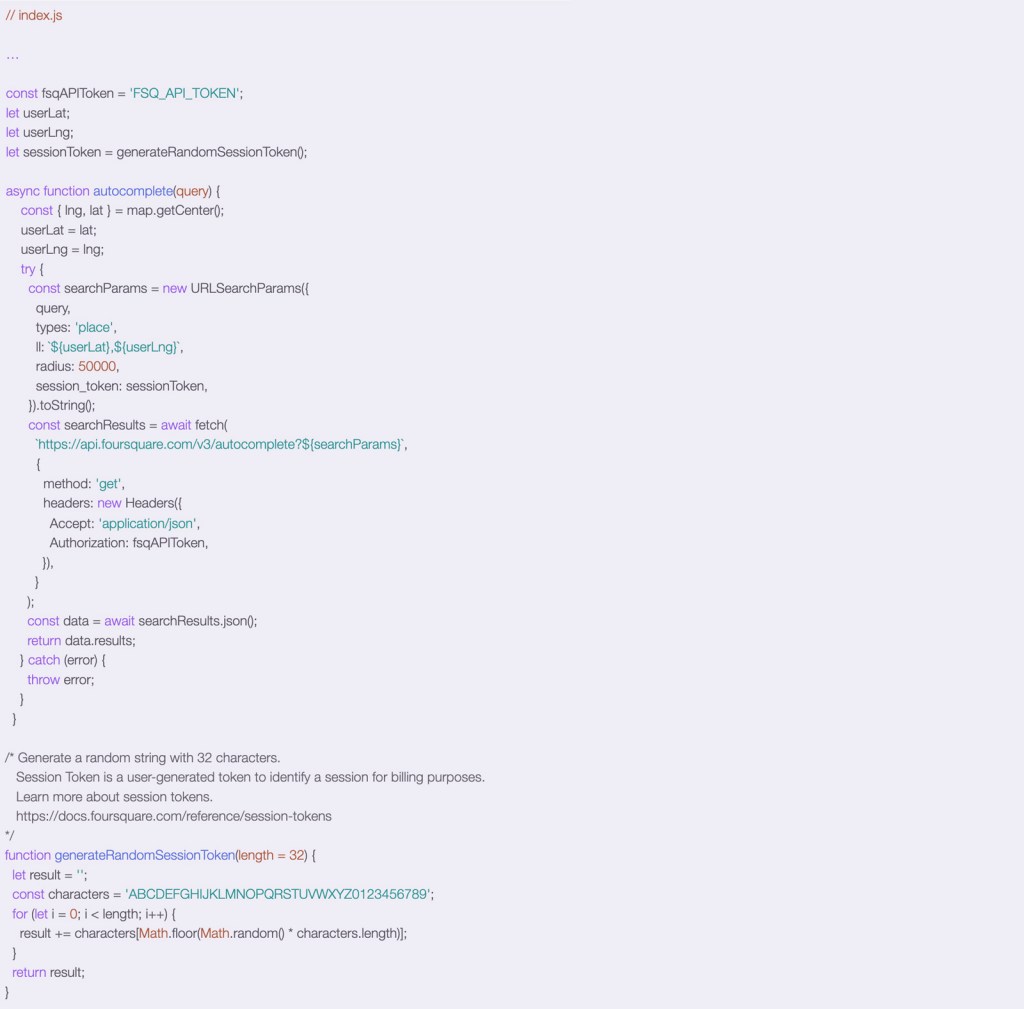
A few callouts:
- The types parameter represents the types of results to return. It can be: address, geo, or place. We will use types: ‘place’ in this example.
- The ll parameter represents the latitude/longitude around which you wish to retrieve the place information.
- The radius parameter defines the distance (in meters) within which to return place results
- The sesstion_token is a user-generated token utilized to group the user query and the user’s selected result into a discrete session for billing purposes. (Note that if the session_token parameter is omitted, the API calls are charged per keystroke/request.) In the example above, we generated a random session token when we were starting to make a request. We’ll show you the complete usage afterwards.
For more information about the query parameters, check out this documentation.
Integrating the search input field
We will now integrate the search input field using the autocomplete method so that we can then add the results to the explorer-suggestions we made before.
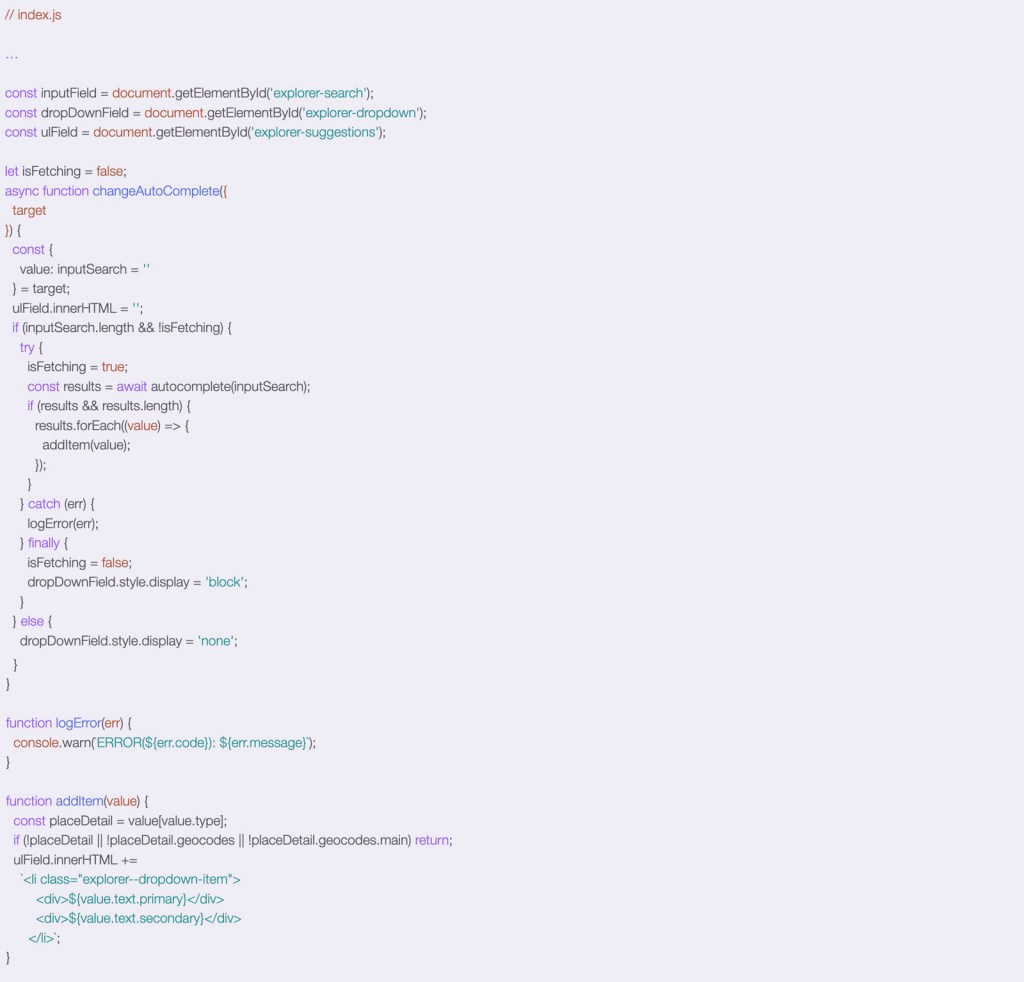
We can see that when we search for something on the search bar, the list of results will appear below.

This is exactly what we want – these are the basics of making a Foursquare autocomplete API call.
Selecting a specific location
Let’s now select an item (or place) and enable the map to center on that place’s location.
1 . Add an onClick event to the < li> item in the addItem method.
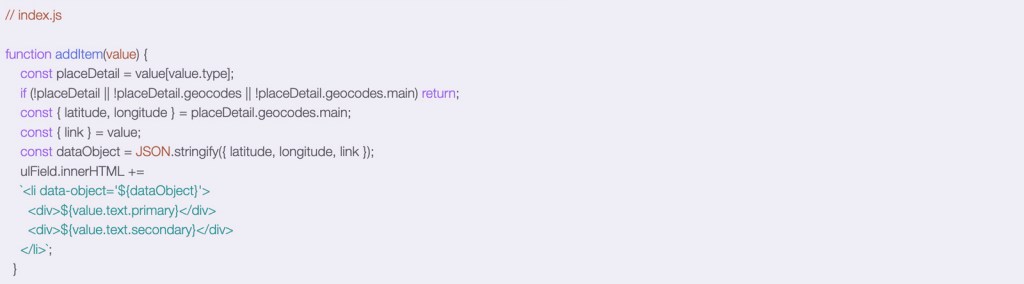
2 . Now you can add the onClick event. Note that we are generating a new session token after a complete search.
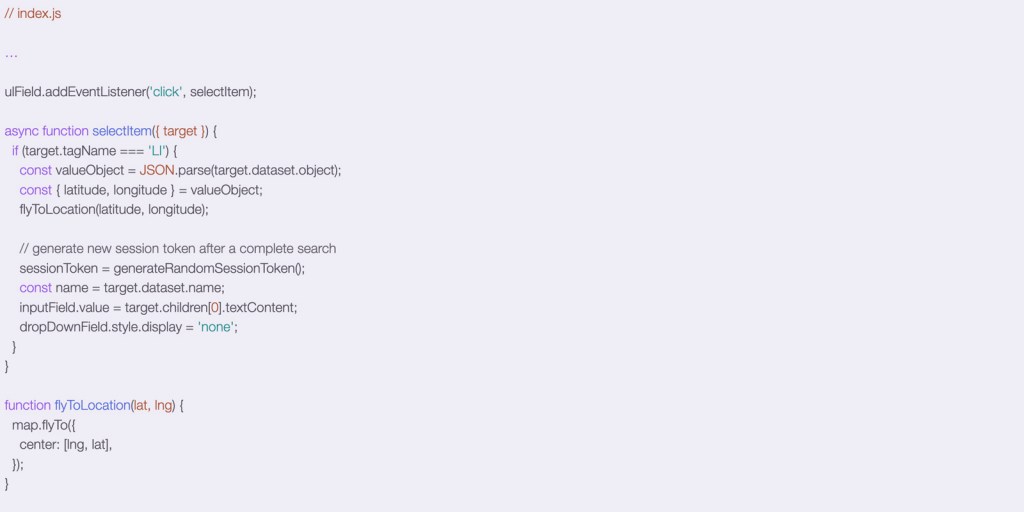
3 . We also need to disable the pointer event for the div inside the < li> item in the CSS file.

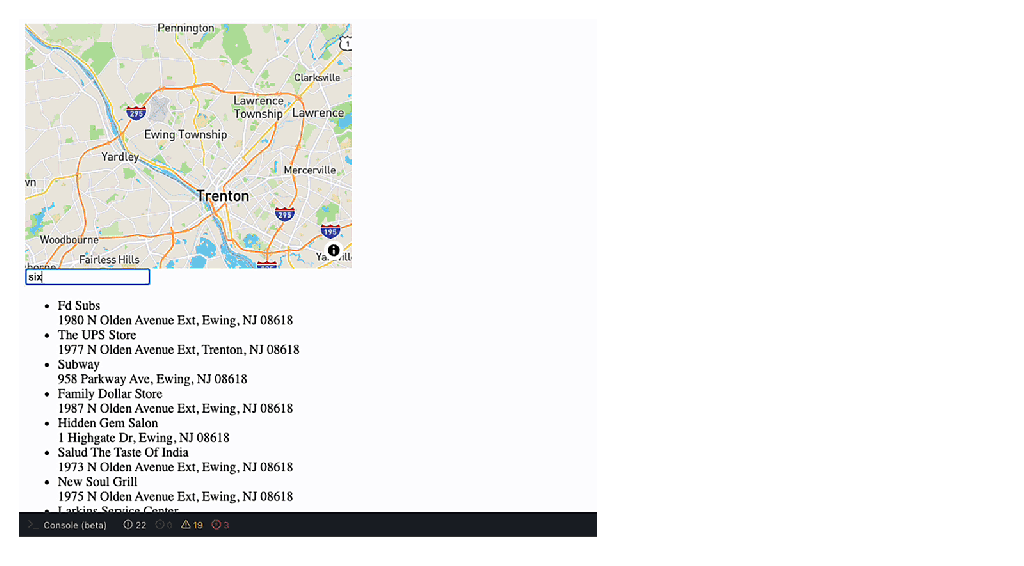
4 . Now, in order to show the place’s details, we have to pin the location on the actual map. To do so, we need to make an additional API call to the Foursquare Places Details endpoint – and luckily, the Foursquare Autocomplete API responses make it very easy to do that. In fact, the response value link gives you the exact path of the place’s details endpoint.
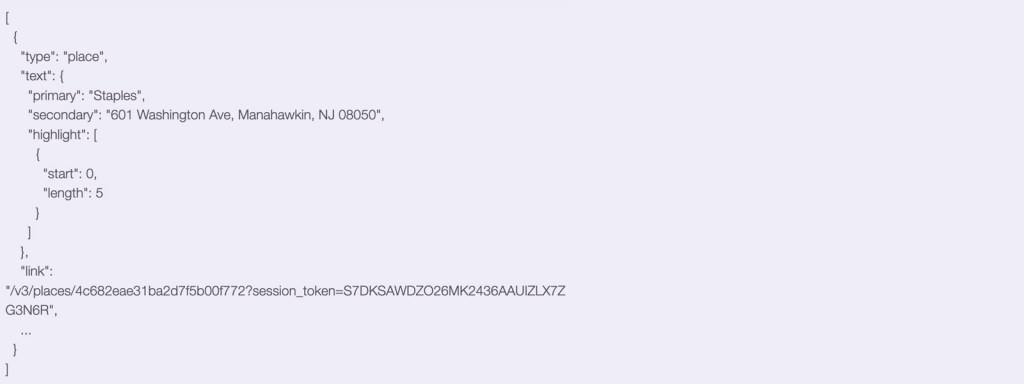
5 . Make another Fetch API call with the link provided by the response.
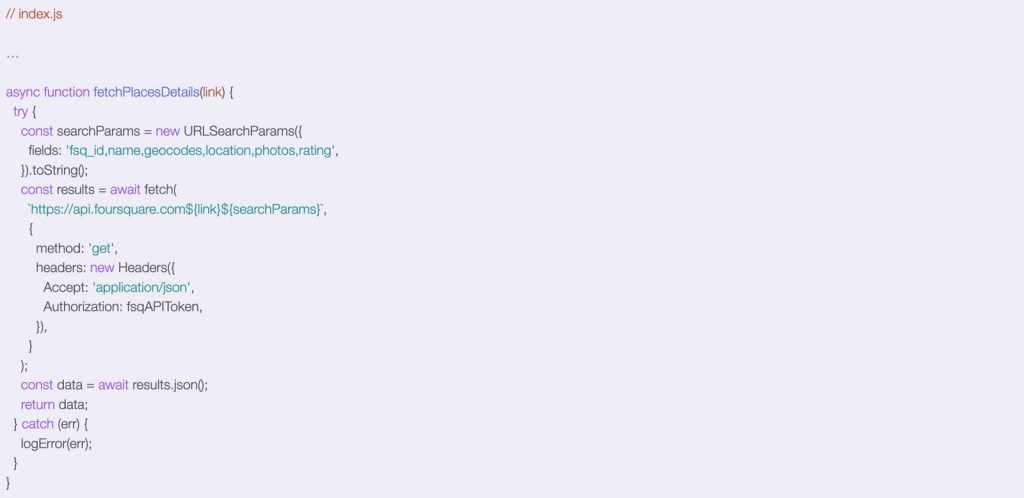
6 . Lastly, we want to add the pin and place’s detail as pop-ups on the map – see below.
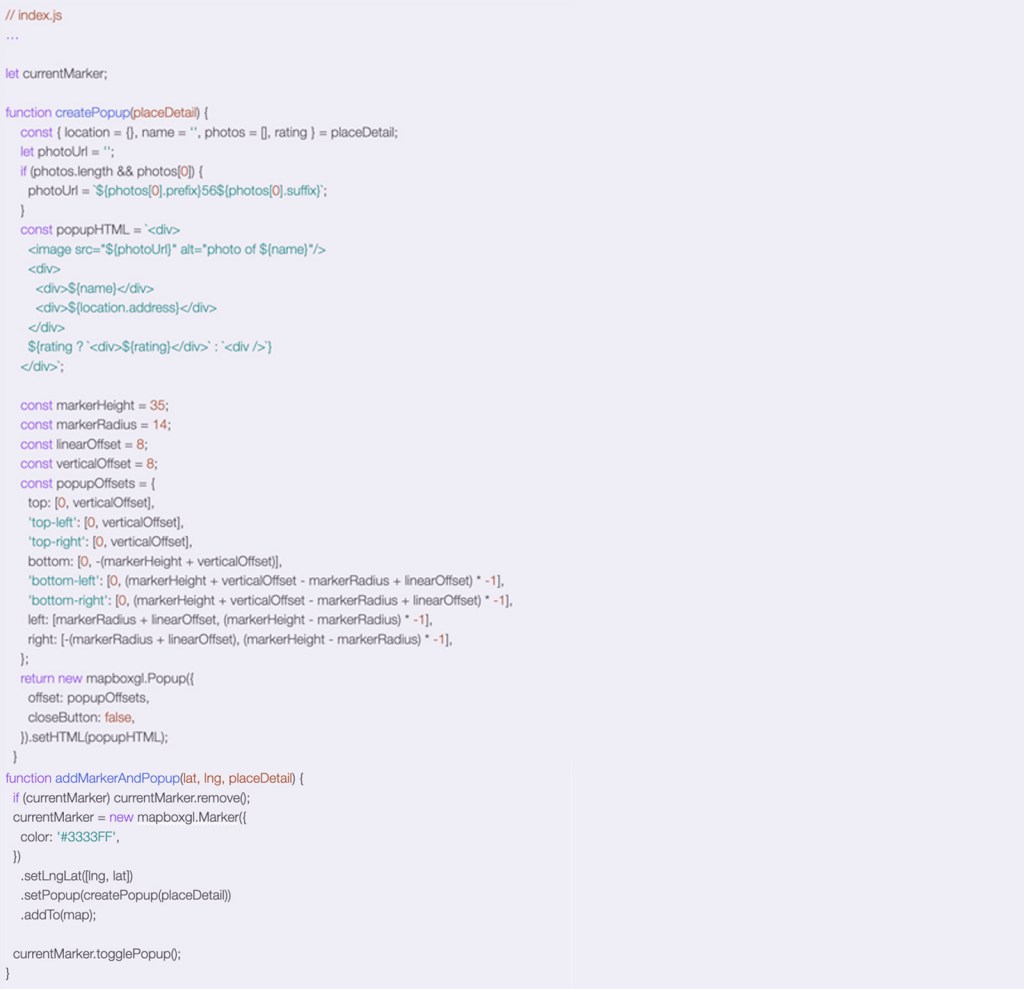
Voilà – we have now completed a simple autocomplete place search on a map.
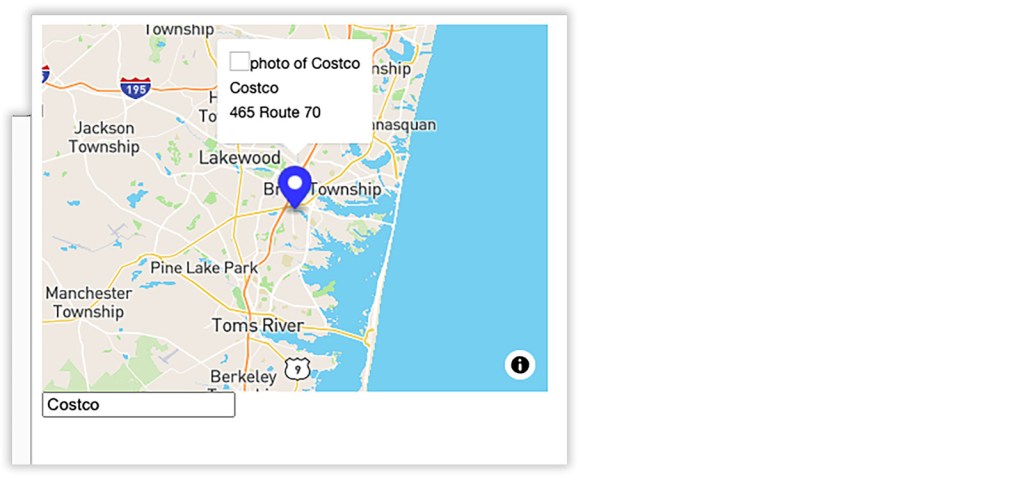
You can access our live demo of this here and additionally refer to the Foursquare documentation page for a more in-depth look.
Want to build this out yourself? Sign up to get started with $200 worth of API calls.